Brute Force Technique: Understanding and Implementing in JavaScript
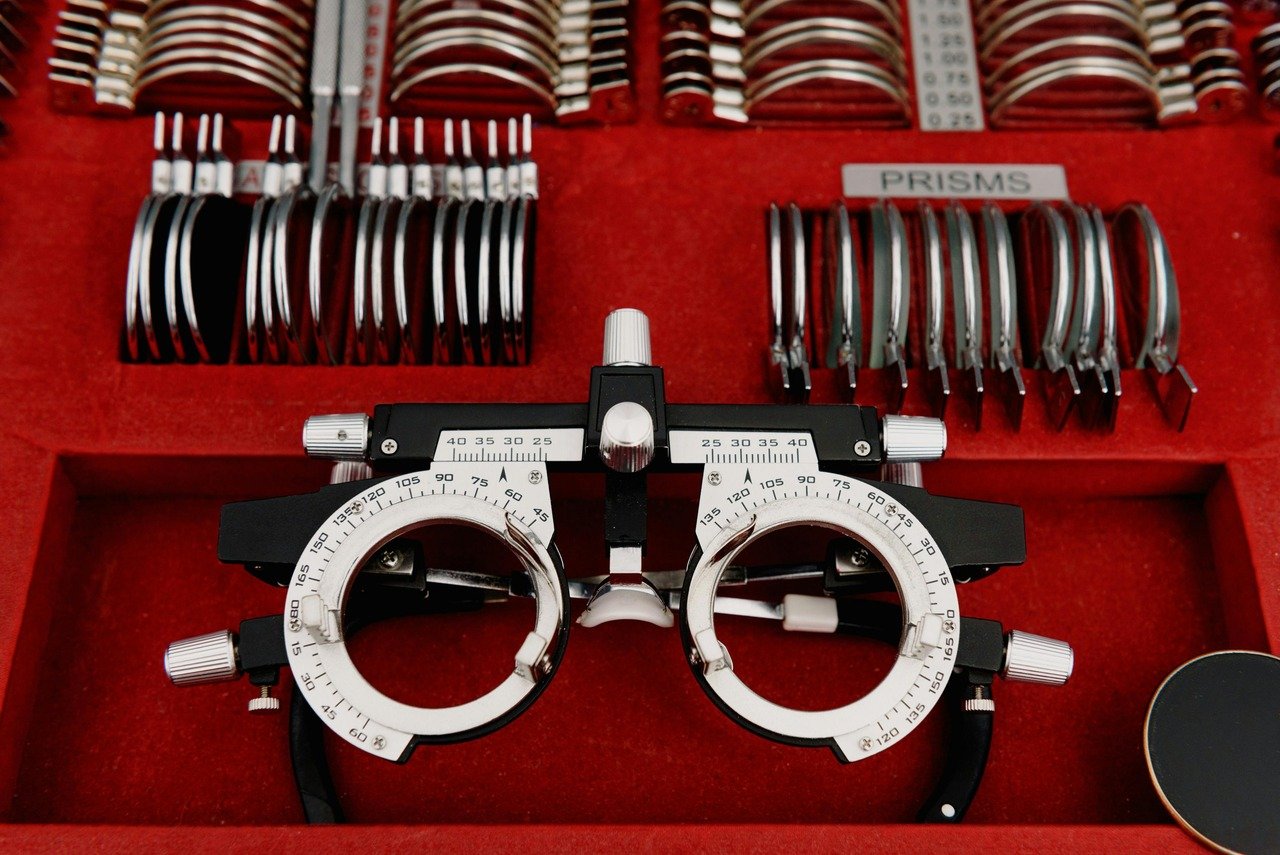
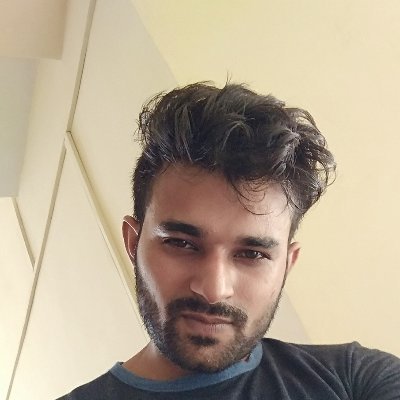
Dr Arun Kumar
PhD (Computer Science)Table of Index
- Understanding Brute Force Technique
- Example: Searching for an Element in an Array
- Problem Statement:
- Brute Force Approach:
- Analysis
- Conclusion
- What is the brute force approach in JavaScript?
- Which algorithm is used in brute force?
- What is the brute force match algorithm?
- What is brute force with example?
- What is brute force algorithm dictionary?
- What is the formula for the brute force algorithm?
- What is better than brute force algorithm?
- Is brute force a greedy algorithm?
- What is an example of a brute force algorithm in real life?
- Which programming language is best for brute force?
- How fast is the brute force algorithm?
Step by Step Example
Frequently Asked Questions
Brute force is a fundamental problem-solving technique used in computer science. It involves systematically checking all possible solutions to a problem until the correct one is found. While not always the most efficient method, brute force can be effective for solving small-scale problems or for situations where other algorithms are not feasible.
In this article, we'll delve into the concept of brute force, its applications, and demonstrate its implementation using JavaScript. We'll use a classic example of finding a specific element in an array to illustrate how brute force works.
Understanding Brute Force Technique
Brute force is essentially a trial-and-error method where all possible solutions are examined systematically. It works by generating all possible candidates and checking each one to see if it satisfies the problem's conditions. While this approach guarantees correctness, it can be inefficient, especially for large problem instances, as it may require examining a vast number of candidates.
Despite its inefficiency, brute force has its merits. It's straightforward to implement and can serve as a baseline for more sophisticated algorithms. Additionally, for small problem sizes or situations where performance is not critical, brute force may be a suitable approach.
Example: Searching for an Element in an Array
Let's consider a simple problem: finding a specific element in an array. We'll use this problem to illustrate how brute force works in practice.
Problem Statement:
Given an array of integers arr and a target value target, find the index of the target value in the array. If the target is not found, return -1.
Brute Force Approach:
The brute force approach to solving this problem involves iterating through each element in the array and comparing it with the target value until a match is found.
Here's how we can implement the brute force solution in JavaScript:
function findIndex(arr, target) {
for (let i = 0; i < arr.length; i++) {
if (arr[i] === target) {
return i; // Found the target, return its index
}
}
return -1; // Target not found
}
// Example usage:
const array = [2, 4, 6, 8, 10];
const targetValue = 6;
console.log(`Index of ${targetValue}:`, findIndex(array, targetValue)); // Output: Index of 6: 2
In this implementation:
- We define a function findIndex that takes an array arr and a target value target.
- We iterate through each element of the array using a for loop.
- Within the loop, we compare each element with the target value.
- If a match is found, we return the index of the element.
- If no match is found after iterating through the entire array, we return -1 to indicate that the target value is not present in the array.
Analysis
While the brute force solution works correctly and is easy to understand, it may not be the most efficient approach, especially for large arrays. The time complexity of the brute force solution for searching an element in an array is O(n), where n is the number of elements in the array. This means that as the size of the array increases, the time taken to search for an element also increases linearly.
Conclusion
Brute force is a straightforward problem-solving technique that involves systematically checking all possible solutions until the correct one is found. While not always the most efficient approach, it can be effective for solving small-scale problems or situations where other algorithms are not feasible.
In this article, we explored the concept of brute force, its applications, and demonstrated its implementation using JavaScript with an example of searching for an element in an array. By understanding the brute force technique, you can gain insights into its strengths and limitations, helping you choose the most appropriate algorithm for solving different problems.
Step By Step Example
Related Questions
What is the brute force approach in JavaScript?
The brute force approach in JavaScript involves solving problems by trying all possible combinations or options until the correct one is found. It is simple but can be inefficient for large inputs as it does not use any optimization techniques. Common examples include searching through arrays, solving puzzles like the traveling salesman problem, or generating permutations of a string.
Which algorithm is used in brute force?
Brute force algorithms include: Linear Search: Iterates through each element in an array to find a target value. Bubble Sort: Repeatedly compares and swaps adjacent elements to sort an array. Password Cracking: Tries all possible combinations until the correct password is found.
What is the brute force match algorithm?
The brute force match algorithm, also known as the naive string matching algorithm, checks for a substring by comparing it sequentially with all possible substrings of the main string. It has a time complexity of O(n*m), where n is the length of the main string and m is the length of the substring.
What is brute force with example?
Brute force refers to solving a problem by exhaustively searching all possible solutions. For example, finding a password by trying every possible combination of characters until the correct one is found is a brute force method. Another example is the brute force approach to the traveling salesman problem, where all possible routes are examined to find the shortest one.
What is brute force algorithm dictionary?
A brute force algorithm dictionary is a method where every possible combination of characters is generated and checked against a hash or password to find the correct one. This method is often used in password cracking, where all potential passwords are tried until the correct one is found.
What is the formula for the brute force algorithm?
The brute force algorithm doesn't have a specific formula but generally involves checking each possible solution until the correct one is found. For example, the time complexity formula for a brute force string matching algorithm is O(n*m), where n is the length of the text and m is the length of the pattern.
What is better than brute force algorithm?
Algorithms better than brute force include: Binary Search: More efficient than linear search for sorted data. Dynamic Programming: Breaks problems into subproblems and stores results to avoid redundant work. Greedy Algorithms: Make optimal local choices to find a global optimum. Divide and Conquer: Breaks problems into smaller subproblems, solves them, and combines results.
Is brute force a greedy algorithm?
No, brute force is not a greedy algorithm. Brute force tries all possible solutions without considering efficiency, whereas a greedy algorithm makes the most optimal choice at each step, aiming for a locally optimal solution that may lead to a globally optimal solution.
What is an example of a brute force algorithm in real life?
A real-life example of a brute force algorithm is the "forgotten password" scenario where you try all possible combinations of characters until the correct password is found. Another example is solving a Rubik's Cube by trying every possible move combination until it is solved.
Which programming language is best for brute force?
Any programming language can be used for brute force algorithms. Popular choices include: Python: Easy syntax and powerful libraries. C++: Fast execution and control over memory. JavaScript: Widely used for web applications and easy to implement. Java: Good for large-scale and complex brute force algorithms.
How fast is the brute force algorithm?
The speed of a brute force algorithm depends on the problem size and complexity. Generally, brute force algorithms are slow for large inputs due to their exhaustive search nature. Their time complexity is often exponential (e.g., O(2^n) for combinatorial problems) or polynomial (e.g., O(n*m) for string matching), making them impractical for large datasets.