Practical Machine Learning with Python
Practical Machine Learning with Python
Introduction
Machine learning (ML) is a subset of artificial intelligence that focuses on the development of algorithms capable of learning and improving from experience without being explicitly programmed. Python, a versatile and widely-used programming language, has become the de facto standard for ML due to its simplicity, rich ecosystem of libraries, and active community. This essay delves into practical aspects of machine learning with Python, guiding readers through foundational concepts, tools, techniques, and real-world applications.
Foundations of Machine Learning
What is Machine Learning?
At its core, machine learning involves the use of data to train algorithms to make predictions or decisions. ML models can be broadly categorized into three types:
-
Supervised Learning: Models are trained on labeled data, where the input-output relationship is known. Examples include regression and classification tasks.
-
Unsupervised Learning: Models identify patterns in data without labeled outcomes. Examples include clustering and dimensionality reduction.
-
Reinforcement Learning: Models learn to make decisions by interacting with an environment to maximize rewards.
Why Python for Machine Learning?
Python’s popularity in ML stems from:
-
Extensive Libraries: Libraries like NumPy, pandas, scikit-learn, TensorFlow, and PyTorch provide prebuilt functions for data manipulation, model building, and evaluation.
-
Ease of Use: Its readable syntax enables rapid prototyping and experimentation.
-
Community Support: Python has a vast and active community contributing to its development and troubleshooting.
Setting Up Your Environment
Python Installation
To start with ML in Python, install Python from its official website or use a package manager like Anaconda, which bundles Python with essential libraries.
Key Libraries
-
NumPy: For numerical computations and array manipulations.
-
pandas: For data manipulation and analysis.
-
Matplotlib & Seaborn: For data visualization.
-
scikit-learn: For ML algorithms and preprocessing.
-
TensorFlow & PyTorch: For deep learning applications.
Integrated Development Environments (IDEs)
Popular IDEs for ML include Jupyter Notebook, PyCharm, and Visual Studio Code. Jupyter Notebook is particularly favored for its interactive features and ease of visualization.
The ML Workflow
1. Data Collection
Data is the backbone of any ML project. Sources can include CSV files, databases, APIs, or web scraping. Python libraries like requests
, BeautifulSoup
, and selenium
aid in web scraping, while SQLAlchemy
connects to databases.
2. Data Preprocessing
Real-world data is often messy and requires cleaning and transformation.
-
Handling Missing Values: Use pandas’
fillna()
ordropna()
methods. -
Feature Scaling: Normalize data using
StandardScaler
from scikit-learn. -
Encoding Categorical Variables: Convert categorical data into numerical using one-hot encoding or label encoding.
3. Exploratory Data Analysis (EDA)
EDA involves summarizing the data to uncover patterns and insights. Visualization tools like Matplotlib and Seaborn help in:
-
Plotting distributions (e.g., histograms).
-
Visualizing correlations using heatmaps.
-
Identifying outliers using box plots.
4. Feature Engineering
Feature engineering enhances the predictive power of models:
-
Feature Selection: Choose the most relevant features using techniques like Recursive Feature Elimination (RFE).
-
Feature Extraction: Create new features using domain knowledge or dimensionality reduction techniques like Principal Component Analysis (PCA).
5. Model Building
-
Choosing an Algorithm:
-
Regression: Linear Regression, Ridge, Lasso.
-
Classification: Logistic Regression, Decision Trees, Support Vector Machines (SVM).
-
Clustering: K-Means, DBSCAN.
-
-
Model Training: Use the
fit()
method to train models on datasets.
6. Model Evaluation
Evaluate models using metrics like:
-
Regression: Mean Squared Error (MSE), R-squared.
-
Classification: Accuracy, Precision, Recall, F1-score.
Tools like cross-validation and hyperparameter tuning improve model reliability.
7. Model Deployment
Deploy models using Flask, Django, or cloud platforms like AWS and Google Cloud.
Practical Examples
1. Predicting House Prices (Supervised Learning)
-
Load and preprocess the dataset using pandas.
-
Perform EDA to understand features like location, size, and price.
-
Train a regression model (e.g., Random Forest) using scikit-learn.
-
Evaluate performance using RMSE.
-
Deploy using Flask for user interaction.
2. Customer Segmentation (Unsupervised Learning)
-
Use a retail dataset containing purchase histories.
-
Preprocess data and scale features.
-
Apply K-Means clustering to segment customers.
-
Visualize clusters using PCA and Seaborn.
3. Image Classification (Deep Learning)
-
Use TensorFlow or PyTorch to build a Convolutional Neural Network (CNN).
-
Train on datasets like MNIST or CIFAR-10.
-
Evaluate using accuracy and confusion matrices.
-
Save the model and deploy it using TensorFlow Serving.
Challenges in Machine Learning
-
Data Quality: Poor data quality leads to unreliable models.
-
Overfitting: Addressed through regularization and cross-validation.
-
Interpretability: Complex models like deep neural networks are harder to interpret.
-
Scalability: Handling large datasets requires optimized tools and infrastructure.
Advancements in Machine Learning
-
AutoML: Automates the ML pipeline from data preprocessing to model deployment.
-
Federated Learning: Enables training models on decentralized data.
-
Explainable AI (XAI): Tools like SHAP and LIME improve model transparency.
-
Integration with IoT: Real-time ML applications in devices like smart assistants.
Take aways
Practical machine learning with Python is an exciting field combining theoretical knowledge with real-world problem-solving. By leveraging Python’s extensive ecosystem, practitioners can efficiently build, evaluate, and deploy ML models. As the field evolves, staying updated with advancements and honing skills through hands-on projects will ensure success in the ML domain.
Latest Posts
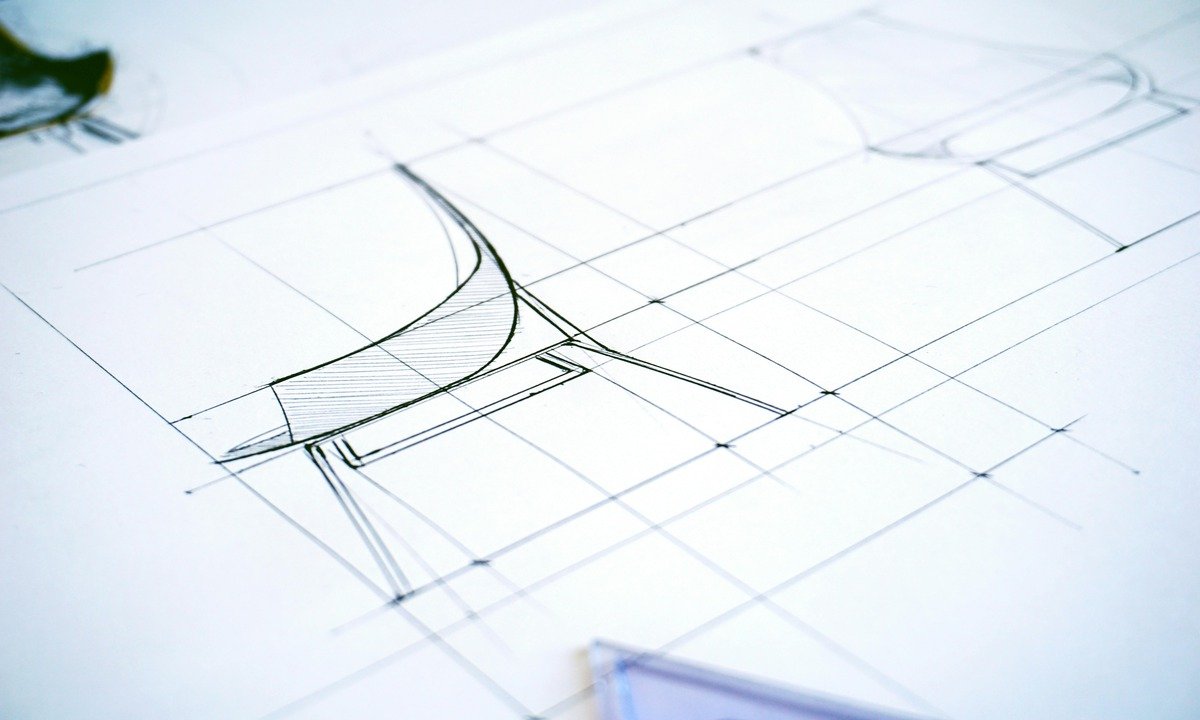
Difference between Qualitative and Quantitative Research with Example
Research methodologies can be broadly categorized into qualitative and quantitative approaches. This article explores these differences using an example, including the use of statistics.
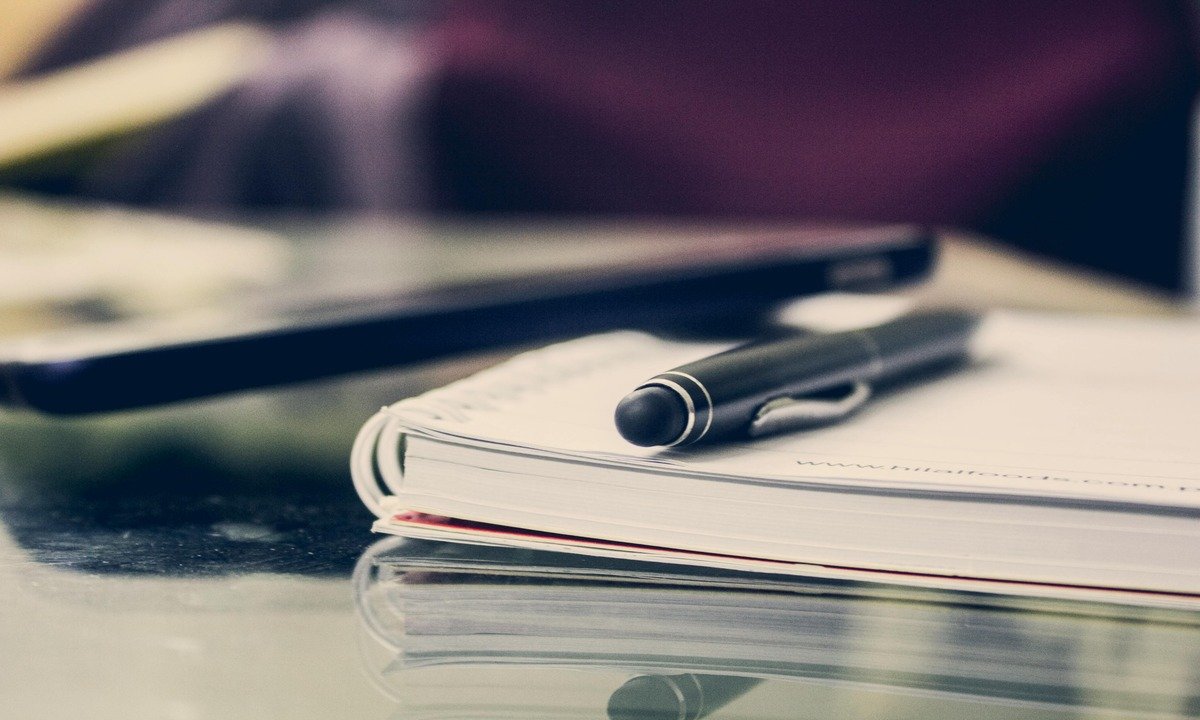
What is Qualitative Research Methodology, Methods and Steps
This comprehensive guide delves into the key aspects of qualitative research methodologies, supported by an example and insights into the qualitative research process.
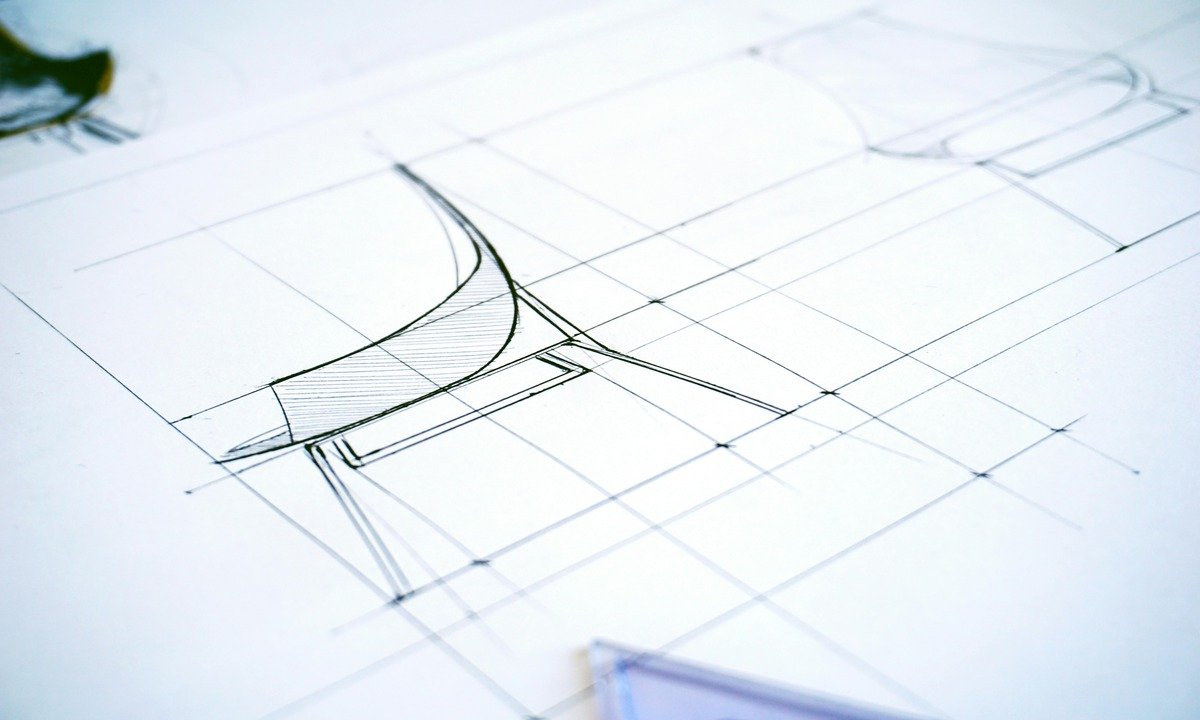
Prim's Algorithm: Understanding Minimum Spanning Trees
Prim's Algorithm is a greedy algorithm used to find the Minimum Spanning Tree (MST) of a weighted, undirected graph.
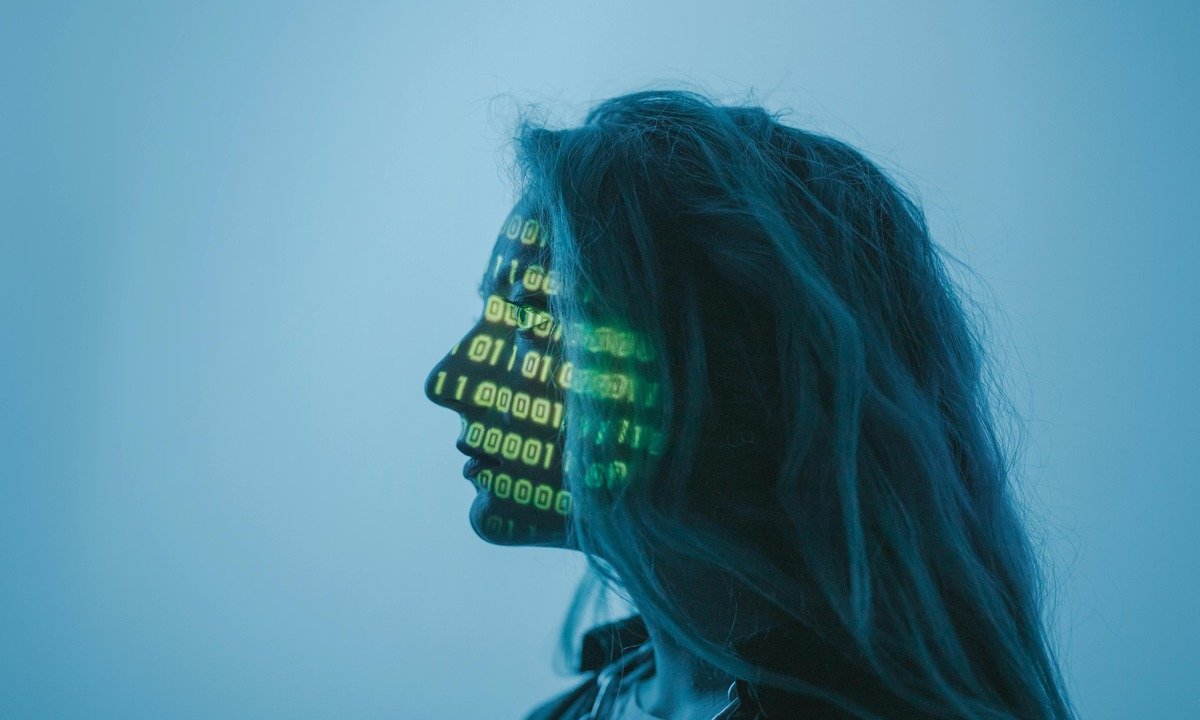
Huffman Coding Algorithm Tutorial
Huffman Coding is a widely used algorithm for lossless data compression. It assigns variable-length codes to input characters, with shorter codes assigned to more frequent characters.
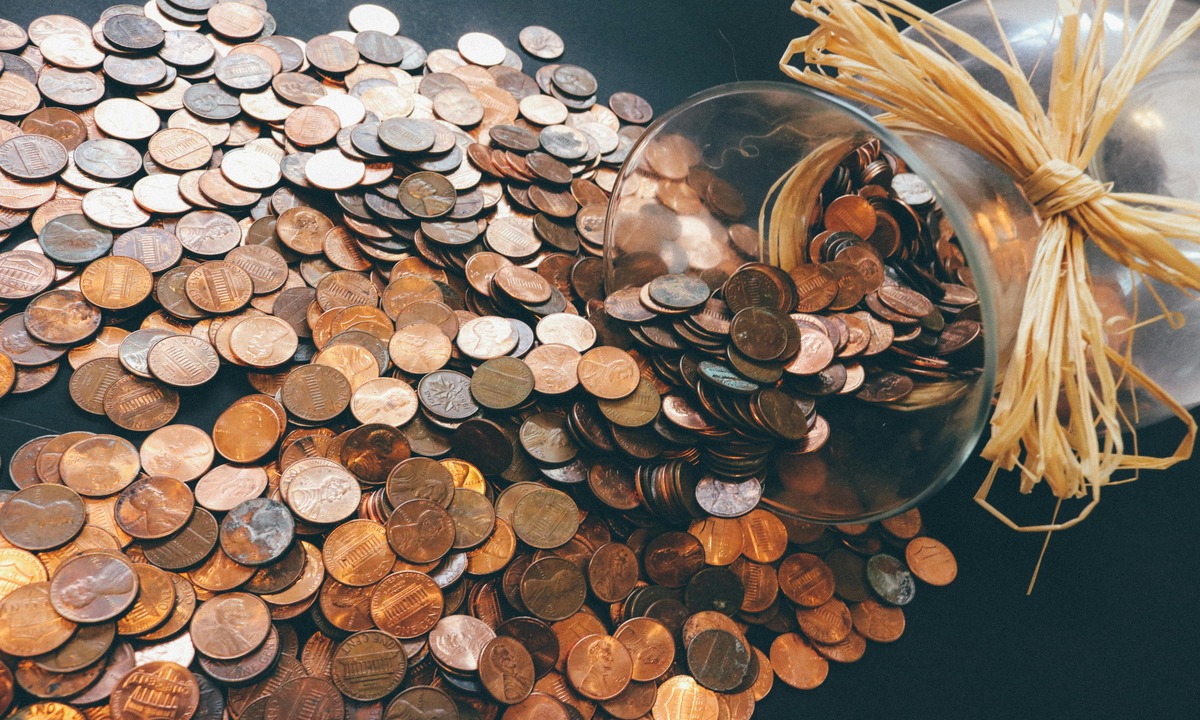
A step by step approach to learn Greedy Algorithm - Data Structure and Algorithms
A greedy algorithm is an approach for solving problems by making a sequence of choices, each of which looks best at the moment.
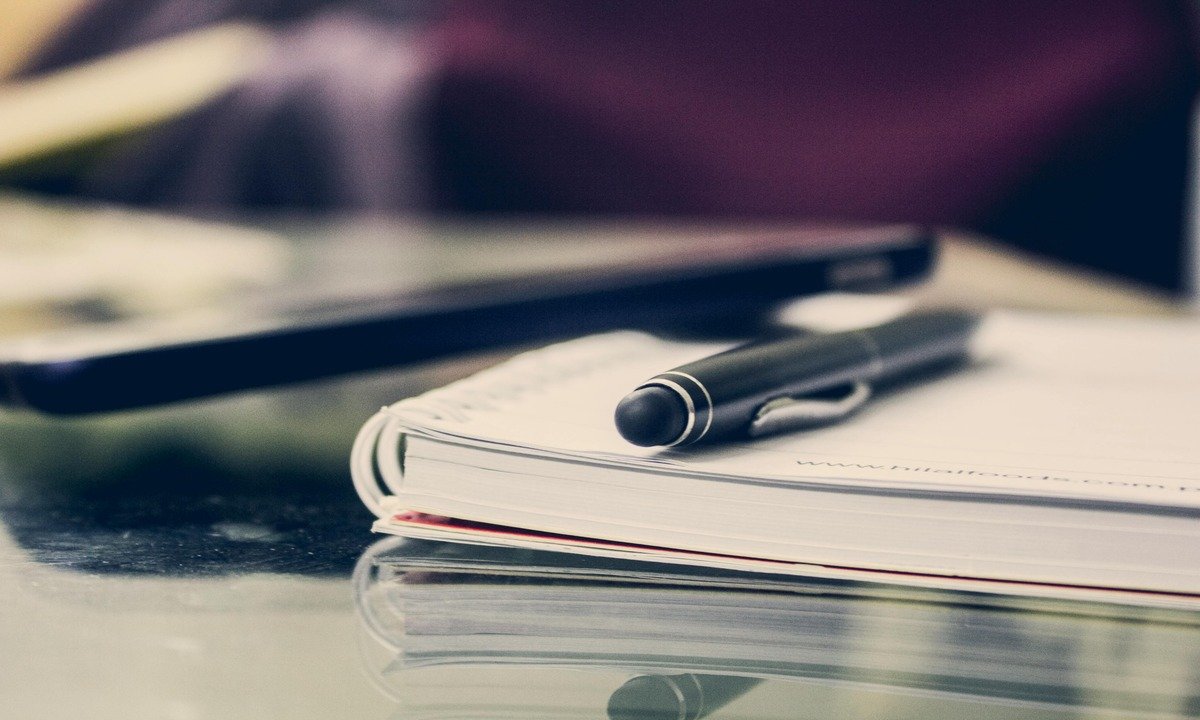
How to write an APA-style research proposal for PhD Admission
Writing a research proposal in APA (American Psychological Association) style involves adhering to specific formatting guidelines and organizational structure.
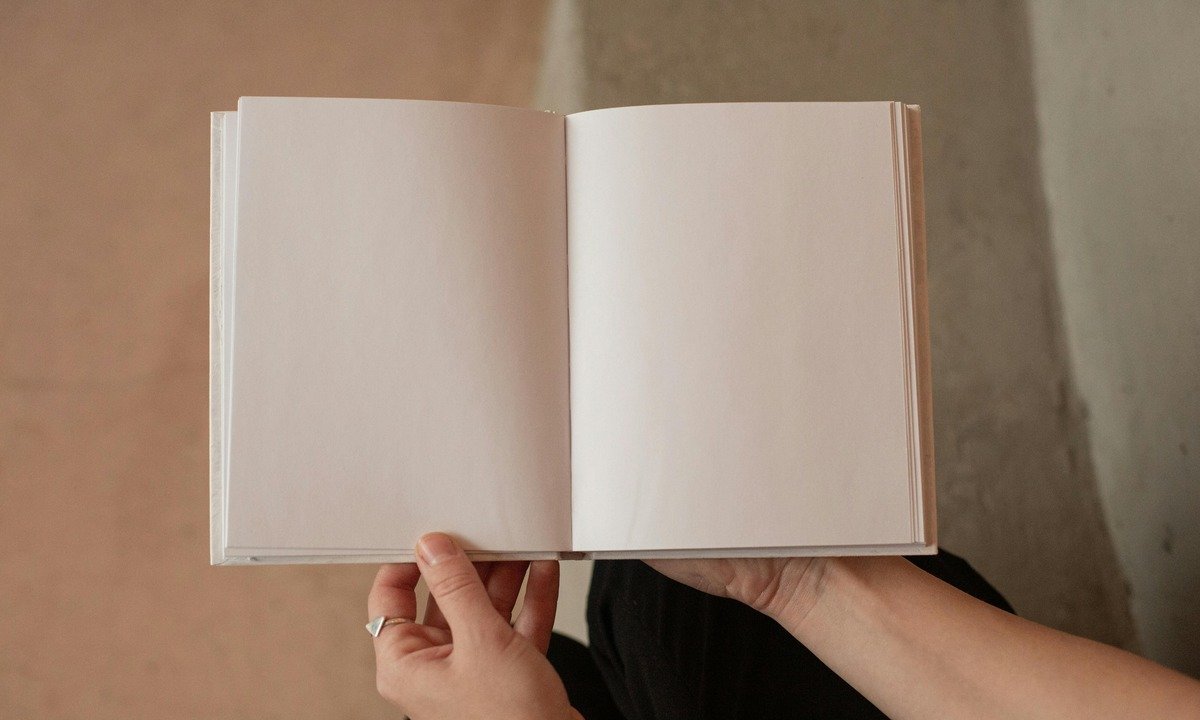
25 steps for Writing a Research Proposal: From Doctoral Research Proposals to Grant Writing and Project Proposals
In this How to write a research proposal guide, we break down the process of writing a research proposal into 25 detailed sections.
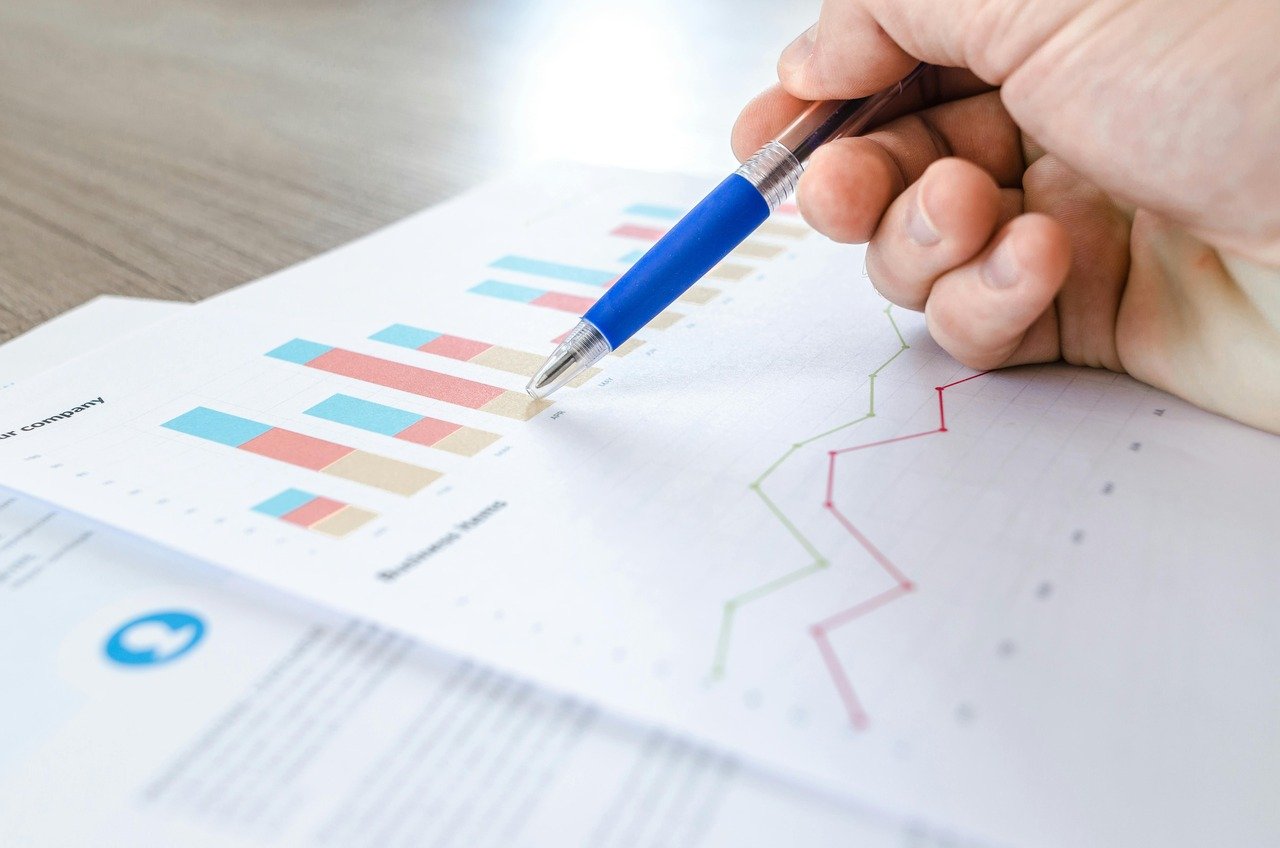
Mastering Linear Regression: A Comprehensive Guide to Data Collection and Analysis for Predictive Modeling
This article provides a comprehensive guide to mastering linear regression, focusing on data collection and analysis.
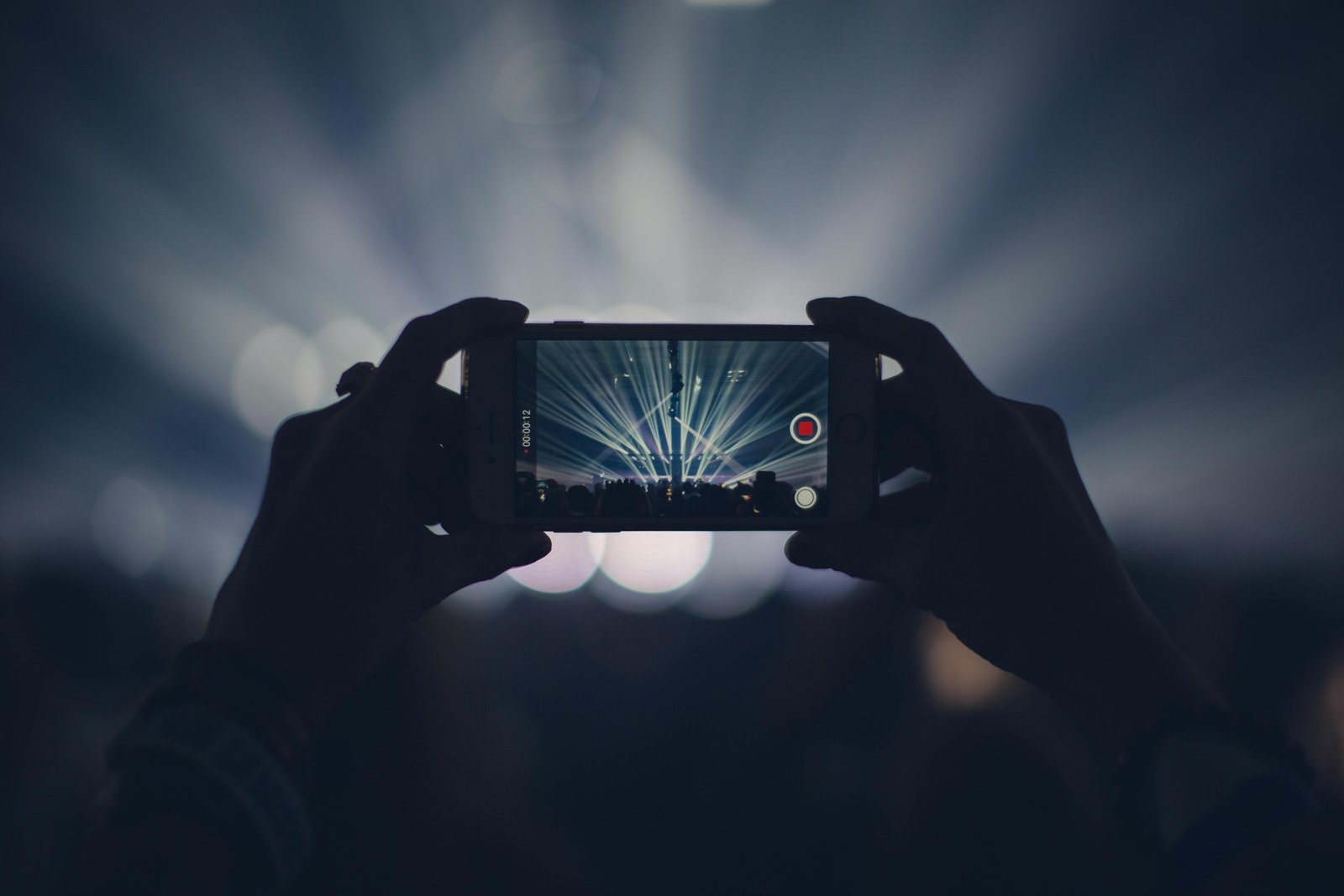
Apple Unveils Groundbreaking AI Innovations at WWDC 2024: Introducing Apple Intelligence and Siri's ChatGPT Integration
Apple's WWDC 2024 introduces Apple Intelligence, revolutionizing AI integration with smarter Siri, ChatGPT capabilities, and innovative features across iOS, iPadOS, and MacOS for enhanced user experience.
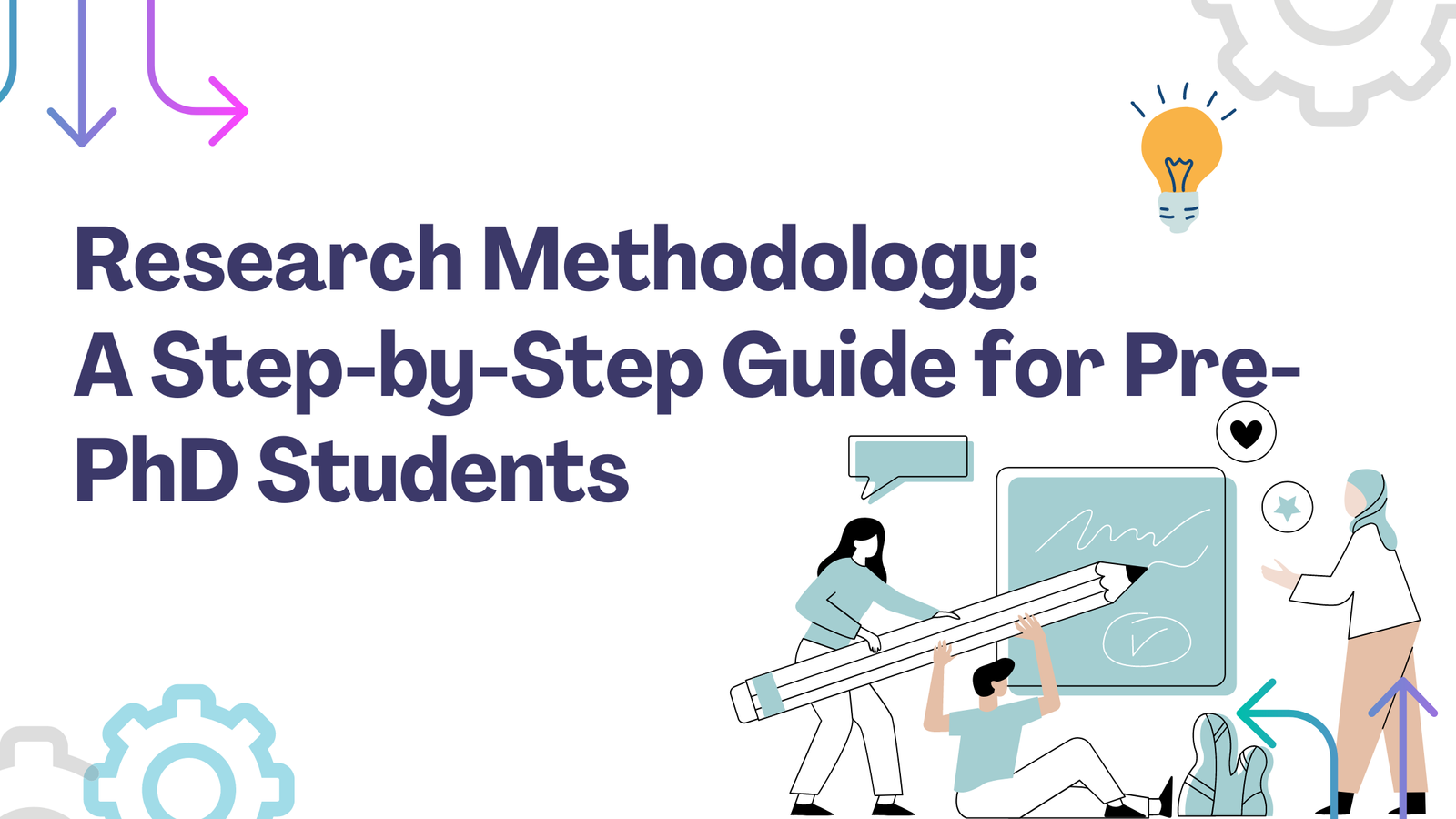
Research Methodology: A Step-by-Step Guide for Pre-PhD Students
research is a journey of discovery, and each step you take brings you closer to finding answers to your research questions.